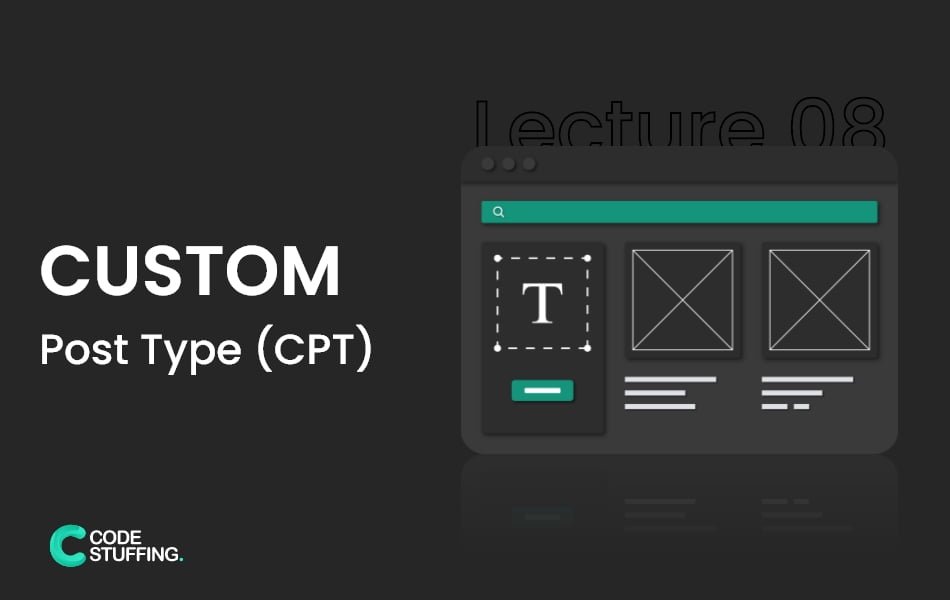

In this lecture, we are going to talk about custom post type, and we will also learn about how to implement them. Let’s put it in a real-world situation. Now we are going to create a medical equipment custom post type.
Before moving further we should know about the difference between post and page.
Page
Page is used for static content, where mostly dynamic recent posts and relative content updates depend on your website. The most important page doesn’t have archives.
Post
Post is used for dynamic post content, where most post details show depending on that post. Post always has archives.
CPT Registration Function
Here is the function to register post type for healthcare and medical equipment, just copy below code and paste it into your functions.php file.
// First Custom Post Type function cpt_equipment(){ $args = array( 'labels' => array( 'name' =>'Equipments', 'singular_name' => 'Equipment', ), 'public' => true, 'has_archive' => true, 'hierarchical'=>true, 'menu_icon'=>'dashicons-heart', 'supports' => array('title','editor','thumbnail'), ); register_post_type('equipment',$args); } add_action('init','cpt_equipment');
Taxonomy Function
After post type, It’s time to create custom taxonomy for our post type with the name of Equipment Type or Type.
Taxonomy is a method that specified data in WordPress. Copy below code and paste it into functions.php file same as above.
// First Taxonomy function tax_equipment_type(){ $args = array( 'labels' => array( 'name' => 'Types', 'singular_name' => 'Types', ), 'public' => true, 'hierarchical' => true, ); register_taxonomy( 'type', array('equipment'), $args); } add_action('init','tax_equipment_type');
Get Custom Taxonomy
Add below code in single.php file to get custom taxonomy that we created.
<?php $equ_tax = get_the_terms( get_the_ID(), 'type' ); ?> <?php if(!empty($equ_tax)):?> <?php foreach ( $equ_tax as $type):?> <li class="d-flex align-items-center mb-2"> <i class='bx bx-folder fs-xl text-muted me-2 pe-1'></i> <a href="<?php echo get_term_link( $type->term_id);?>"> <?php echo $type->name; ?> </a> </li> <?php endforeach; ?> <?php endif; ?>
Permalink Update
After creating Custom Post Type and Custom Taxonomy, The most important thing is to update Settings -> Permalink, and we will use this post type as a unique functionality.
#BeingCodeStuffer